PyClaw Solutions¶
PyClaw Solution
objects are containers for
State
and Domain
objects
that define an entire solution.
The State
class is responsible for containing all
the data of the solution on the given Domain
.
The Domain
is responsible for containing
the geometry of the Solution
. The structure of a
solution may look something like the figure.
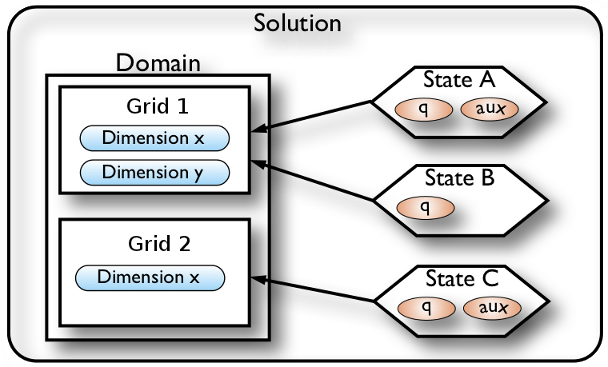
Pyclaw solution structure including a Domain
,
a set of Patch
objects and corresponding
Dimension
objects defining the solution’s
geometry and three State
objects pointing to
the appropriate Patch
with varying fields.¶
List of serial and parallel objects in a Solution
class:
Serial |
Parallel |
---|---|
|
|
|
|
|
|
|
|
|
pyclaw.solution.Solution
¶
-
class
clawpack.pyclaw.solution.
Solution
(*arg, **kargs)¶ Pyclaw patch container class
- Input and Output
Input and output of solution objects is handle via the io package. Solution contains the generic methods
write()
,read()
andplot()
which then figure out the correct method to call. Please see the io package for the particulars of each format and method and the methods in this class for general input and output information.- Properties
If there is only one state and patch belonging to this solution, the solution will appear to have many of the attributes assigned to its one state and patch. Some parameters that have in the past been parameters for all patch,s are also reachable although Solution does not check to see if these parameters are truly universal.
- Patch Attributes:
‘dimensions’
- State Attributes:
‘t’,’num_eqn’,’q’,’aux’,’capa’,’problem_data’
- Initialization
The initialization of a Solution can happen one of these ways
args is empty and an empty Solution is created
args is an integer (the number of components of q), a single State, or a list of States and is followed by the appropriate geometry object which can be one of:
(
Domain
)(
Patch
) - A domain is created with the patch or list of patches provided.(
Dimension
) - A domain and patch is created with the dimensions or list of dimensions provided.
args is a variable number of keyword arguments that describes the location of a file to be read in to initialize the object
- Examples
>>> import clawpack.pyclaw as pyclaw >>> x = pyclaw.Dimension('x',0.,1.,100) >>> domain = pyclaw.Domain((x)) >>> state = pyclaw.State(domain,3,2) >>> solution = pyclaw.Solution(state,domain)
-
is_valid
()¶ Checks to see if this solution is valid
The Solution checks to make sure it is valid by checking each of its states. If an invalid state is found, a message is logged what specifically made this solution invalid.
- Output
(bool) - True if valid, false otherwise
-
plot
()¶ Plot the solution
-
read
(frame, path='./_output', file_format=None, file_prefix='fort', read_aux=True, options={}, **kargs)¶ Reads in a Solution object from a file
Reads in and initializes this Solution with the data specified. This function will raise an IOError if it was unsuccessful.
Any format must conform to the following call signiture and return True if the file has been successfully read into the given solution or False otherwise. Options is a dictionary of parameters that each format can specify. See the ascii module for an example.:
read_<format>(solution,path,frame,file_prefix,options={})
<format>
is the name of the format in question.- Input
frame - (int) Frame number to be read in
path - (string) Base path to the files to be read.
default = './_output'
file_format - (string) Format of the file, should match on of the modules inside of the io package.
default = None
but now attempts to read from header file (as of v5.9.0).file_prefix - (string) Name prefix in front of all the files, defaults to whatever the format defaults to, e.g. fort for ascii
options - (dict) Dictionary of optional arguments dependent on the format being read in.
default = {}
- Output
(bool) - True if read was successful, False otherwise
-
set_all_states
(attr, value, overwrite=True)¶ Sets all member states attribute ‘attr’ to value
- Input
attr - (string) Attribute name to be set
value - (id) Value for attribute
overwrite - (bool) Whether to overwrite the attribute if it already exists.
default = True
-
write
(frame, path='./', file_format='ascii', file_prefix=None, write_aux=False, options={}, write_p=False)¶ Write out a representation of the solution
Writes out a suitable representation of this solution object based on the format requested. The path is built from the optional path and file_prefix arguments. Will raise an IOError if unsuccessful.
- Input
frame - (int) Frame number to append to the file output
path - (string) Root path, will try and create the path if it does not already exist.
default = './'
format - (string or list of strings) a string or list of strings containing the desired output formats.
default = 'ascii'
file_prefix - (string) Prefix for the file name. Defaults to the particular io modules default.
write_aux - (book) Write the auxiliary array out as well if present.
default = False
options - (dict) Dictionary of optional arguments dependent on which format is being used.
default = {}
-
property
patch
¶ (
Patch
) - Base state’s patch is returned
-
property
start_frame
¶ (int) - : Solution start frame number in case the Solution object is initialized by loading frame from file
-
property
state
¶ (
State
) - Base state is returned